C++ is a powerful programming language that is used extensively in the development of complex software applications. One of the key features of C++ is its support for templates, which enable developers to write generic code that can be used with different data types. The Standard Template Library (STL) is a set of C++ template classes that provide commonly used data structures and algorithms. In this article, we will explore the STL in detail, looking at its history, design, and functionality.
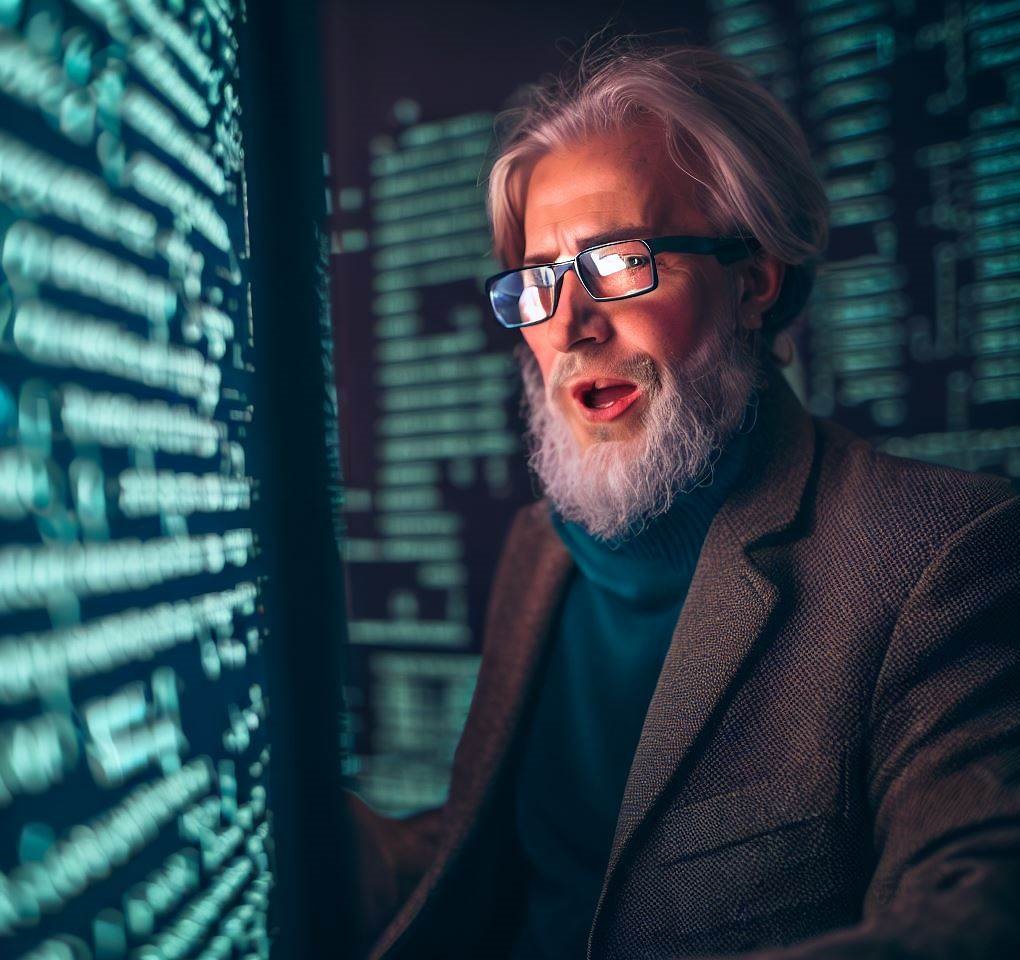
History of the STL
The STL was first proposed in 1990 by Alexander Stepanov, a researcher at Hewlett-Packard. Stepanov had been developing generic programming techniques for several years, and he saw the potential for a library of reusable data structures and algorithms that could be used with any data type. He collaborated with David Musser, a researcher at AT&T Bell Labs, and they worked together to create the initial version of the STL.
The first version of the STL was released as part of the C++ Standard Library in 1994, with the publication of the ANSI/ISO C++ standard. Since then, the STL has become an integral part of the C++ programming language, and it is used by millions of developers around the world.
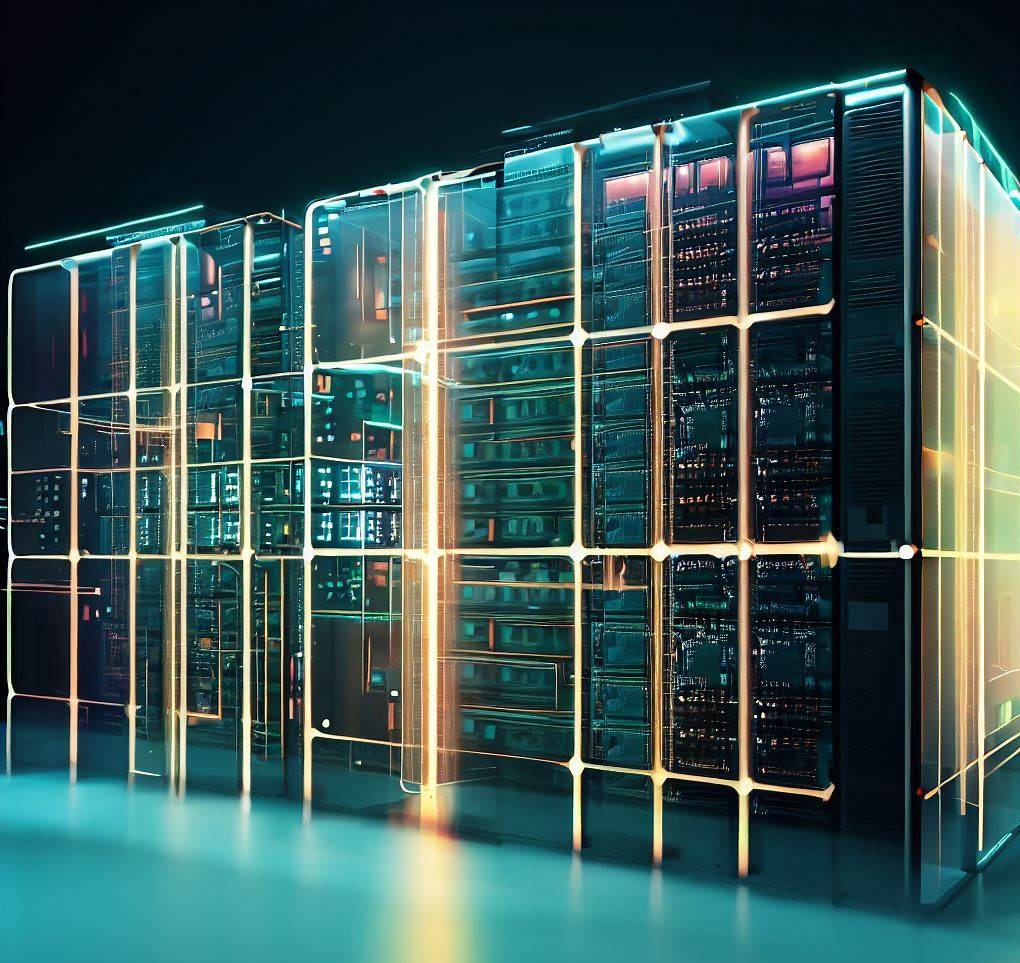
Design of the STL
The STL is designed to be a flexible and efficient library that provides a wide range of data structures and algorithms. It consists of several components, including containers, iterators, algorithms, and function objects.
Containers are classes that hold collections of objects, such as vectors, lists, and maps. They provide a convenient way to store and manipulate data, and they are designed to be efficient and easy to use.
Iterators are classes that provide a way to access the elements of a container. They allow developers to iterate over the elements of a container in a flexible and efficient way, and they can be used with any container class.
Algorithms are functions that operate on containers and perform common operations, such as searching, sorting, and manipulating data. They are designed to be generic and flexible, so they can be used with any container class and any data type.
Function objects are classes that encapsulate a function or a function pointer. They can be used with algorithms to provide custom behavior, such as sorting elements in a specific way or filtering elements based on certain criteria.
The STL is designed to be extensible and customizable, so developers can create their own containers, iterators, algorithms, and function objects. This makes it a powerful tool for building complex software applications.
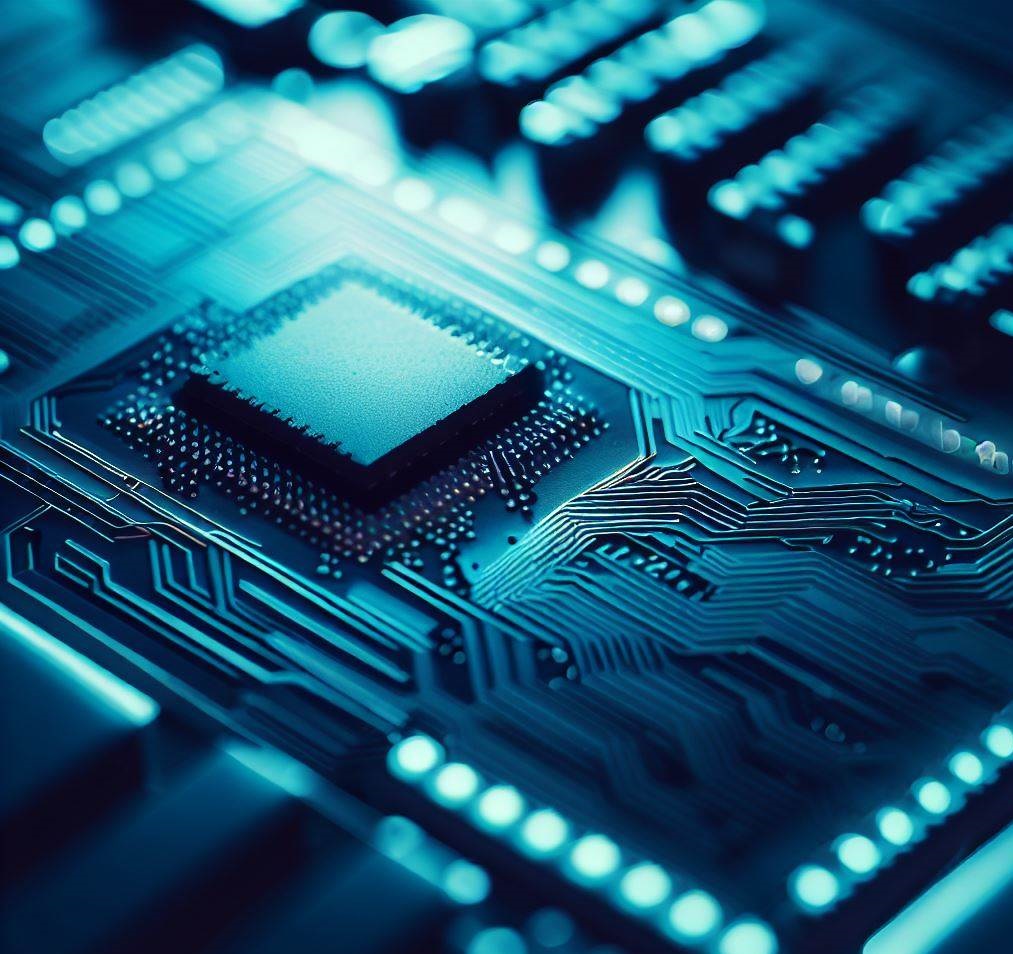
Functionality of the STL
The STL provides a wide range of functionality that makes it a versatile library for developing software applications. Some of the key features of the STL include:
- Containers: The STL provides a variety of container classes, including vectors, lists, sets, maps, and queues. These containers can be used to store and manipulate data in a variety of ways, and they are designed to be efficient and flexible.
- Iterators: The STL provides several types of iterators, including input iterators, output iterators, forward iterators, bidirectional iterators, and random access iterators. These iterators provide a way to access the elements of a container in a flexible and efficient way, and they can be used with any container class.
- Algorithms: The STL provides a wide range of algorithms, including sorting, searching, merging, transforming, and more. These algorithms are designed to be generic and flexible, so they can be used with any container class and any data type.
- Function objects: The STL provides a variety of function objects, including predicates, functors, and binders. These function objects can be used with algorithms to provide custom behavior, such as sorting elements in a specific way or filtering elements based on certain criteria.
- Memory management: The STL provides a way to manage memory efficiently using smart pointers. Smart pointers are objects that behave like pointers, but they automatically manage the memory they point to. This makes it easy to avoid memory leaks and other common memory-related issues.
- Exception handling: The STL provides a way to handle exceptions that may be thrown during the execution of algorithms or other operations. This makes it easy to write robust and reliable code that can handle unexpected situations gracefully.
- Localization: The STL provides a way to support internationalization and localization in software applications. This allows developers to create software that can be easily adapted to different languages and cultural settings.
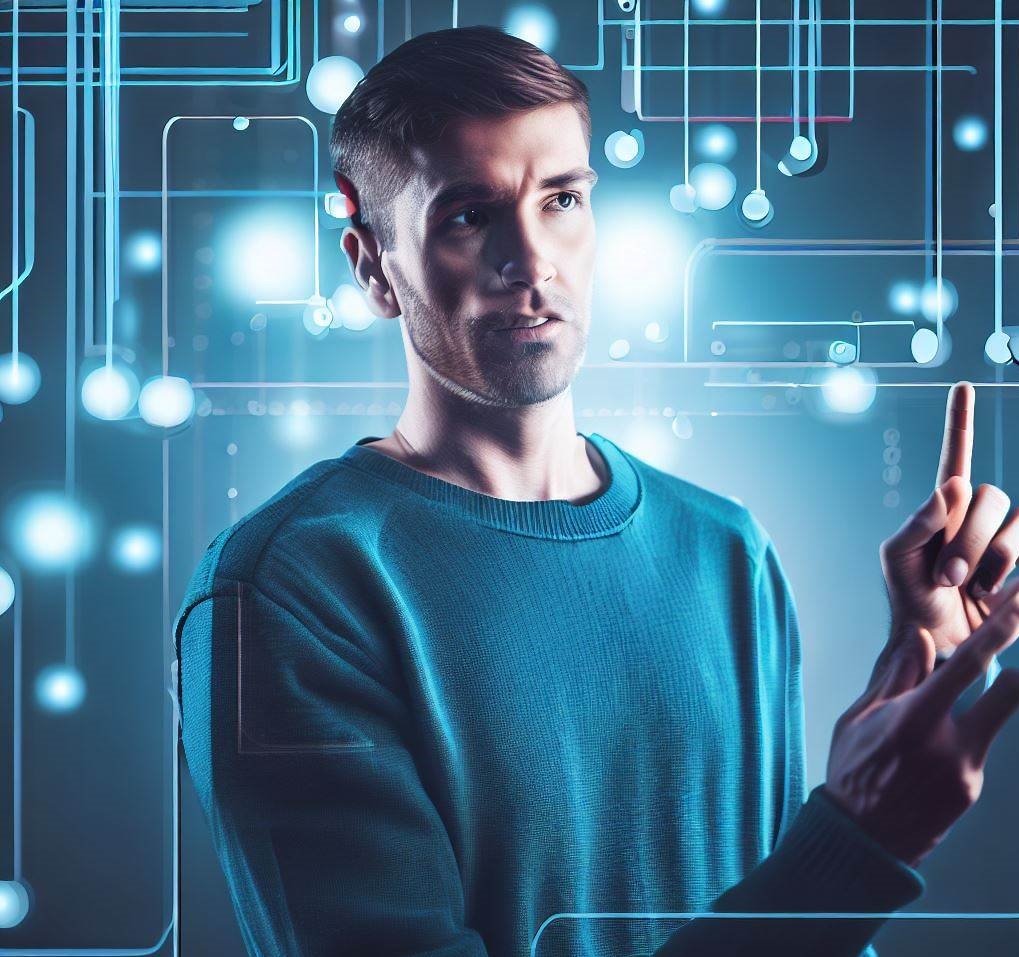
Benefits of Using the STL
The STL provides several benefits for developers, including:
- Reusability: The STL provides a wide range of generic data structures and algorithms that can be easily reused in different software applications. This saves time and effort, as developers do not need to reinvent the wheel every time they write a new program.
- Efficiency: The STL is designed to be efficient, with algorithms and data structures that are optimized for performance. This makes it ideal for developing software applications that require high performance and low latency.
- Flexibility: The STL is designed to be flexible, with a wide range of container classes, iterators, algorithms, and function objects that can be customized and extended as needed. This makes it a powerful tool for building complex software applications.
- Standardization: The STL is part of the C++ Standard Library, which means that it is a widely accepted and standardized library that can be used on any platform that supports C++. This makes it easy to write cross-platform software applications that work on different operating systems and hardware architectures
Conclusion
The Standard Template Library is a powerful and flexible library that provides a wide range of data structures and algorithms for developers. It is designed to be efficient, reusable, and customizable, making it ideal for developing complex software applications. Whether you are building a simple command-line utility or a large-scale enterprise application, the STL is a valuable tool that can help you get the job done quickly and efficiently.